JAVA-10 Short and Float Variables
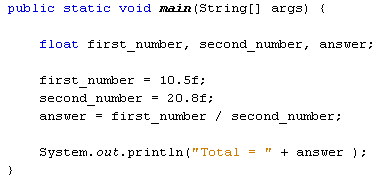
Two more variable types you can use are short and float . The short variable type is used to store smaller number, and its range is between minus 32,768 and plus 32,767. Instead of using int in our code on the previous pages, we could have used short instead. You should only use short if you're sure that the values that you want to hold don't go above 32, 767 or below -32,768. The double value we used can store really big numbers of the floating point variety. Instead of using double, float can be used. When storing a value in a float variable, you need the letter "f" at the end. Like this: float first_number, second_number, answer; first_number = 10.5f; second_number = 20.8f; So the letter "f" goes after the number but before the semicolon at the end of the line. To see the difference between float and double,